2020年12月6日
Jerry
4070
2021年1月17日
前面三篇把升级大体思路直观的通过按钮展示了出来,那这一篇就应用到具体程序中,实现真正的一个程序的升级。
这里的想法还是主程序与升级程序分开实现,流程图如下:
一、升级程序制作
1、新建一个项目,画下面一个界面,将整个流程以进度条形式直观展现:
2、作为升级辅助程序,在程序启动时接受三个参数,分别是主程序检测更新时解析出来的:version(升级版本号) url(升级路径) md5(校验码)
/// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main(string[] args)
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
if (args.Length == 0)
{
//MessageBox.Show("无参数!");
return;
}
else if (args.Length == 3)
{
Application.Run(new Form1(args));
}
else
{
return;
}
}
//Form1.cs
public static string[] g_temp;
public Form1(string[] args)
{
InitializeComponent();
/*
* temp[0] version
* temp[1] url
* temp[2] md5
*/
g_temp = args;
}
3、参数传进来后,在Form1窗体加载最后一步的函数中新启一个进程,实现预先写好的升级流程即可。
/// <summary>
/// 窗体加载最后一步启动进程
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void Form1_Shown(object sender, EventArgs e)
{
Thread thread = new Thread(new ThreadStart(start_Update));//创建线程
thread.Start(); //启动线程
}
/// <summary>
/// 开始升级
/// </summary>
private void start_Update()
{
if (0 != get_File())
{
MessageBox.Show("下载升级文件失败!");
return;
}
if (0 != check_File())
{
MessageBox.Show("升级文件校验失败!");
return;
}
if (0 != unzip_File())
{
MessageBox.Show("升级文件解压失败!");
return;
}
if (0 != copy_File())
{
MessageBox.Show("升级文件复制失败!");
return;
}
label1.Text = "升级成功!正在启动...";
string exename = System.Environment.CurrentDirectory + "\\picbrowser.exe";
WinExec(exename, 1);
Thread.Sleep(1500);
//升级完退出
Application.Exit();
}
二、主程序制作
这里拿之前写过的MD5校验工具做例子,原始版本界面如下,版本号为:1.0.0.0
1、新建一个Update类,实现两个方法:下载update.xml文件和检查版本号
public class Update
{
private string g_upxmlname;
private string g_localxmlname = "local.xml";
public struct MyXml_S
{
public string version;
public string url;
public string md5;
};
public MyXml_S g_upxml;
public MyXml_S g_loxml;
public int get_XML( string url)
{
WebClient client = new WebClient();
try
{
g_upxmlname = System.IO.Path.GetFileName(url);
client.DownloadFile(url, System.Environment.CurrentDirectory + "\\" + g_upxmlname);
return 0;
}
catch (Exception ex)
{
return -1;
}
}
public int parse_XML()
{
try
{
//解析 下载 XML
XmlDocument doc = new XmlDocument();
doc.Load(System.Environment.CurrentDirectory + "\\" + g_upxmlname);
XmlElement root = null;
root = doc.DocumentElement;
XmlNodeList nodeList = null;
nodeList = root.SelectNodes("/Update/Soft");
foreach (XmlElement el in nodeList)//读元素值
{
List<String> strlist = new List<String>();
foreach (XmlNode node in el.ChildNodes)
{
strlist.Add(node.InnerText);
}
g_upxml.version = strlist[0];
g_upxml.url = strlist[1];
g_upxml.md5 = strlist[2];
}
//解析 本地XML
doc.Load(System.Environment.CurrentDirectory + "\\" + g_localxmlname);
root = doc.DocumentElement;
nodeList = root.SelectNodes("/Local/Soft");
foreach (XmlElement el in nodeList)//读元素值
{
List<String> strlist = new List<String>();
foreach (XmlNode node in el.ChildNodes)
{
strlist.Add(node.InnerText);
}
g_loxml.version = strlist[0];
}
if (g_loxml.version == g_upxml.version)
{
//richTextBox1.AppendText("\n无需升级!");
string dstpath = System.Environment.CurrentDirectory;
File.Delete(dstpath + "\\" + g_upxmlname);
return 0;
}
else
{
//richTextBox1.AppendText("\n版本号校验完成,需要升级!");
string dstpath = System.Environment.CurrentDirectory;
File.Delete(dstpath + "\\" + g_upxmlname);
return 1;
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
return -1;
}
}
}
2、主程序启动时下载升级文件update.xml,并检查版本号是否升级。在program.cs中修改代码:
/// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Update update = new Update();
// 填入设置好的升级地址
if (0 != update.get_XML("xxxxxxxxxxxxx"))
{
MessageBox.Show("下载升级文件失败!");
return;
}
int ret = update.parse_XML();
if (-1 == ret)
{
MessageBox.Show("解析升级文件失败!");
return;
}
else if (1 == ret)
{
MessageBox.Show("检查到更新,请点击确认进入升级程序..");
System.Diagnostics.Process pro = new System.Diagnostics.Process();
pro.StartInfo.FileName = System.Environment.CurrentDirectory + "\\up1.0.exe";
//传入4个字符串
pro.StartInfo.Arguments = string.Format("{0} {1} {2}", update.g_upxml.version, update.g_upxml.url, update.g_upxml.md5);
pro.Start();//开启程序
}
else if (0 == ret)
{
Application.Run(new Form1());
}
}
实现的就是程序启动时检查更新,如果需要更新就把获取到的升级参数传递给升级程序“up1.0.exe”,开始升级。
最后把升级文件及升级包的地址准备好就可以开始升级啦!
最后的实现效果:
升级后界面:
原创文章,转载请注明出处:
https://jerrycoding.com/article/csharp-autoupdate4
微信
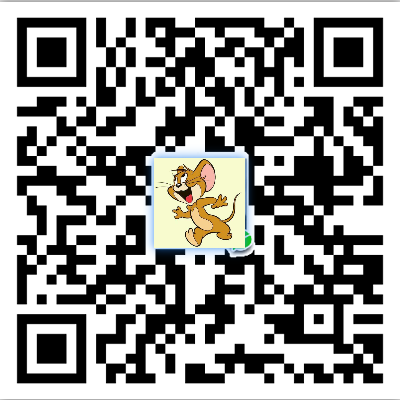
支付宝
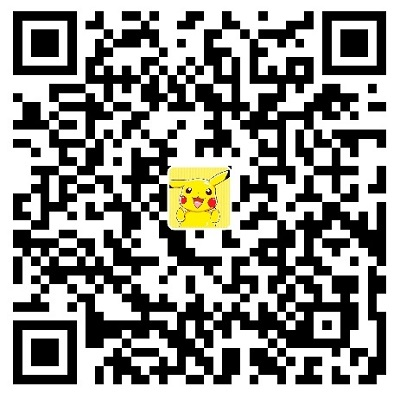